Introduction to Computer Science II
Homework 4
Due by 11:50am on Tuesday, February 1
Reading
Read chapters 8 and 9 and the week 4 lecture notes.
Problems
Solve the following by implementing the corresponding functions in homework4.py, increment.py, and bmi.py. When done, submit
all three files through D2L.
1. Write a container class called
PriorityQueue. The class should support methods:
- insert(n): Takes a number n as input and adds it to
the container
- min(): Returns the smallest number in the container
- removeMin() Removes the smallest number in the
container
- isEmpty(): Returns True if container is empty, False
otherwise
In addition to these methods your class shoudl support the len()
function as shown below.
Usage:
>>> pq = PriorityQueue()
>>> pq.insert(3)
>>> pq.insert(1)
>>> pq.insert(5)
>>> pq.insert(2)
>>> pq.min()
1
>>> pq.removeMin()
>>> pq.min() 2
>>> len(pq)
3
>>> pq.isEmpty()
False
2. Do problem 8.16 in the textbook.
3. Do problem 8.18 in the textbook.
4. Do problem 8.39 in the textbook.
Usage:
>>> dude = Hacker()
>>> snoopy = Dog()
>>> garfield = Cat()
>>> tweety = Animal()
>>> tweety.speak()
>>> garfield.speak()
Meeow!
>>> snoopy.speak()
Wooof!
>>> dude.speak()
Hello World!
>>> kingkong = Primate()
>>> kingkong.speak()
Boooh
5. [Lab] Implement in increment.py a GUI
that has two buttons:
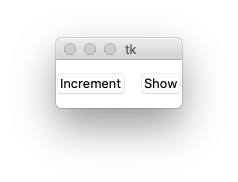
The button labeled Increment
will increment an integer n, initially 0, and the button labeled
Show will show the value of n in a popup window. Hint: Make n a global variable.
Usage:
After starting the GUI, if you were to click the Show button, a popup
window will appear showing the initial value of n:
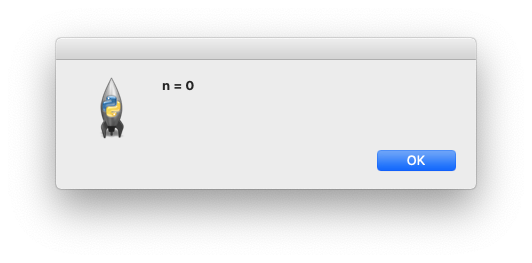
Click OK. Now
suppose you click the Increment
button once and then ckick Show. You will see another popup
window:
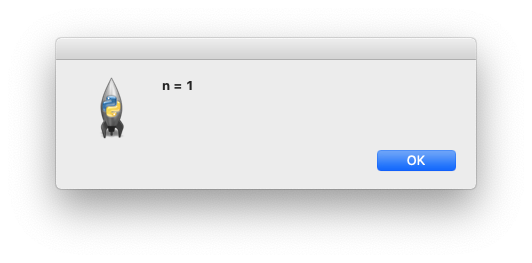
Click OK. Now
suppose you click the Increment
button three times and then ckick Show. You will see another popup
window:
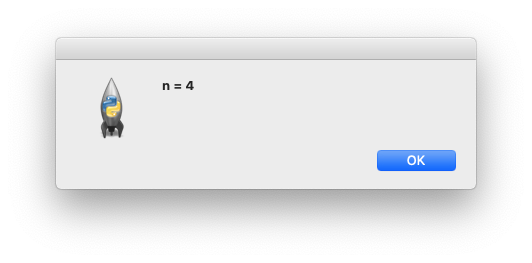
6. [Lab]
Implement in bmi.py an
app that allows users to compute their body mass index (BMI), which
is defined in Practice Problem 5.1 of your textbook. Your GUI should
look as follows:
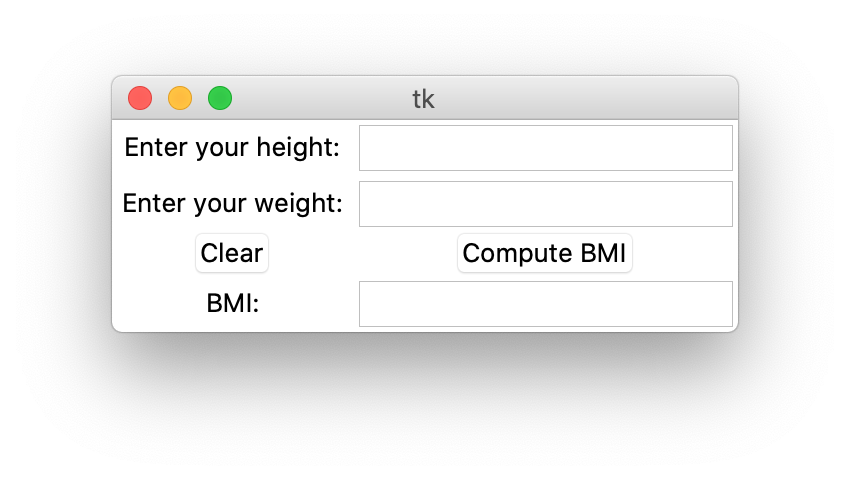
After entering the weight and height and then clicking the button,
your BMI should appear in the Entry labeled BMI:
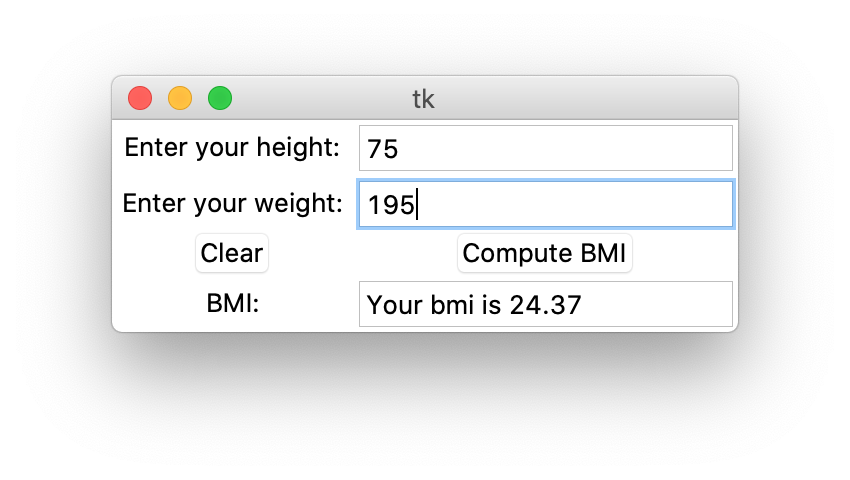
Make sure that your GUI is user friendly by deleting the entered
weight and height so that the user can enter new inputs without
having to erase the old ones.