Introduction to Computer Science II
Winter 2022 Midterm Practice Exam
Solve the below problems by
implementing the appropriate classes and methods in file midtermPractice.py.
Problems
1. [30pts] Write a class named Vehicle that can be used as
shown below:
Usage:
>>> v = Vehicle()
>>> v.add_cargo('train')
>>> v.add_cargo('ball')
>>> v.add_cargo('doll')
>>> v.cargo_contents()
['train', 'ball', 'doll']
>>> v.deliver()
'doll'
>>> v.cargo_contents()
['train', 'ball']
Note: you will need to implement an __init__ constructor
in addition to methods add_cargo, cargo_contents,
and deliver. Method deliver should remove and
return the last item added to the cargo (i.e., last in,
first out).
2. [30 pts]
Write a class named Sled as a subclass of Vehicle
that inherits all the attributes of Vehicle and also:
- extends the Vehicle
__init__ constructor to take, as input, the coordinates
x and y of the Vehicle object,
- supports method move that takes number values
dx and dy as input and moves
the sled by dx
units along the x-axis and by dy units along the y-axis, and
- overloads the __str__
operator so it behaves as shown below.
Usage:
>>> s = Sled(0,0)
>>>
s.add_cargo('train')
>>>
s.add_cargo('ball')
>>>
s.cargo_contents()
['train', 'ball']
>>> s.move(2,1)
>>> s.deliver()
'ball'
>>> print(s)
Gifts being delivered at
location (2,1)
>>> s.move(3,7)
>>> s.deliver()
'train'
>>> print(s)
Gifts being delivered at
location (5,8)
3. [30 points] Implement a GUI widget
class Calculator that consists of an Entry widget
and a Button widget labeled "Compute":
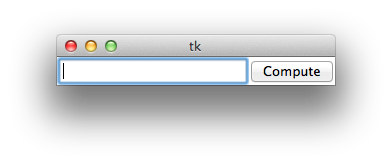
To use the app, the user should enter an arithmetic expression in
the Entry:
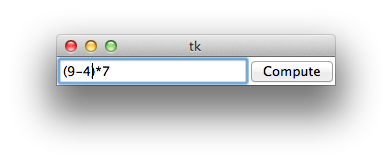
When the user clicks on the Button, a popup window should display
the result:
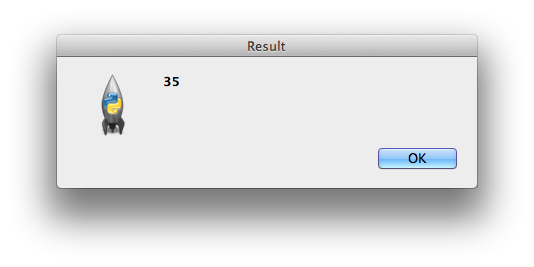
After the user clicks OK in the popup window, the Entry should be
empty and ready for the next expression (as shown in the first
image).
Usage:
>>> Calculator().pack()
4. [10 points] Implement a GUI
widget class App that consists of the Calculator
widget your just implemented and the Draw widget covered in class
(and already included in the midtermPractice.py
file):
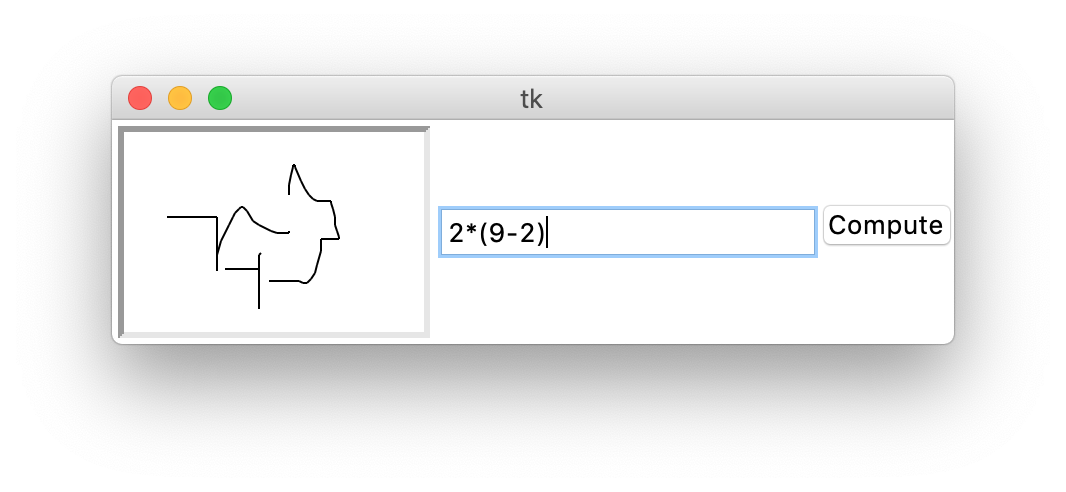
Usage:
>>> App().pack()