Introduction to Computer Science II
Homework 5
Due by 11:50am on Tuesday, February 8
Reading
Read chapter 9 and the week 4 lecture notes.
Midterm exam practice
The midterm exam is scheduled for Thursday, Feb 10. A practice
midterm exam will be assigned during the lab session on Monday,
February 7.
Solutions will be posted on D2L on Monday, right after the end of
the lab.
Problems
Solve the following by implementing the corresponding functions in homework5.py. When
done, submit that file through D2L.
1. When you run the code in clickIt2.py, you
will get a GUI that contains just one Frame widget of size 480
x 640 and that has this behavior: Every time the user clicks
at some location in the frame, the location coordinates are printed
in the interactive shell. Run the prgram and try it.
Now refactor this program into a class ClickIt2. (Note: Do
your work in file homework5.py).
Usage:
>>>
ClickIt2().pack() #
THIS STARTS THE GUI; THE BELOW OUTPUT APPEARS WHEN YOU CLICK IT
>>> you clicked at (98, 81)
you clicked at (200, 169)
you clicked at (258, 130)
you clicked at (549, 311)
you clicked at (323, 412)
you clicked at (215, 286)
2. Implement a GUI widget class BMI that
allows users to compute their body mass index (BMI). The BMI GUI
should behave exactly the same as the BMI GUI in problem 6 of the homework assignment 4.
Feel free to refactor the solution I posted on D2L.
Usage:
>>> BMI().pack()
3. Implement in class Craps a GUI
that simulates the gambling game craps. The GUI should include a
button labelled "New game" that starts a new game by simulating the
initial roll of a pair of dice. The result of the initial roll is
then shown in an Entry widget (as shown below). If the initial roll
is not a win or a loss, the user will have to click the button "Roll
for point", and keep clicking it until she wins.

Usage:
>>> Craps().pack()
4. Develop new GUI widget class Ed
that can be used to teach first-graders addition and subtraction.
The GUI should contain two Entry widgets and a Button widget labeled
"Enter". When started using
Usage:
>>> Ed().pack()
you would see:
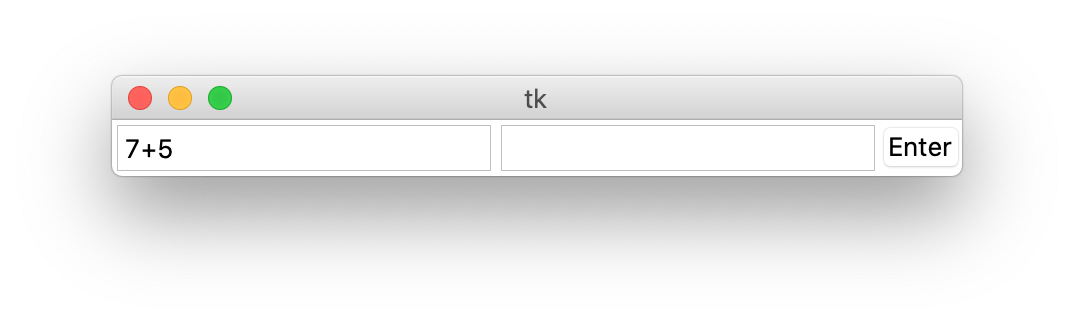
As shown above, at start-up your program should generate (1) two
single-digit pseudorandom numbers a and b and
(2) an operation o, which could be addition or
subtraction—with equal likelihood—using the randrange()
function in the random module. The expression a o b
will then be displayed in the first Entry widget (unless a
is less than b and the operation o is
subtraction, in which case b o a is displayed, so the
result is never negative). Expressions displayed could be, for
example, 3+2, 4+7, 5-2, 3-3 but could not be 2-6. The user will have
to enter, in the second Entry widget, the result of evaluating the
expression shown in the first Entry widget and click the "Enter"
button. If the correct result is entered, a new window should say
"You got it!".
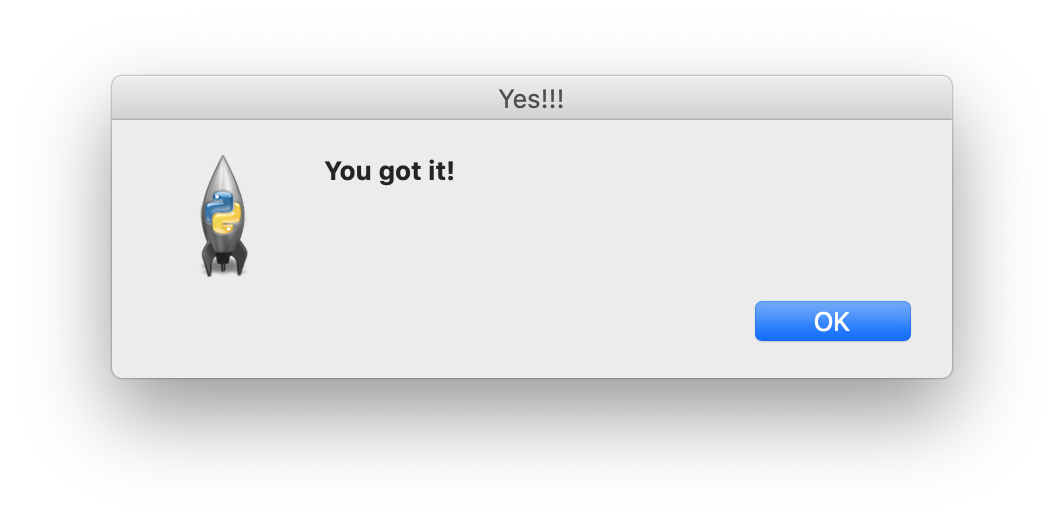
5. Augment the GUI you developed in Problem
4. so that a new problem gets generated after the user
answers a problem correctly. In addition, your app should keep track
of the number of tries for each problem and include that information
in the message displayed when the user gets the problem right.
